Project: Electronics Bash - Arduino #6- Putting it Together
In this stream, we'll be looking at:
- Driving LED Matrices
- Using Shift registers to expand input and output capabilities
- Tying together input, output, and processing into a cohesive game
This is the space where I'll be posting code samples and circuit layouts sometime in advance of the stream, for those who want to follow along more directly. You can expect the code and formatting here to change up to a couple hours before the stream as the plan for the evening comes together.
Shift Register Demos
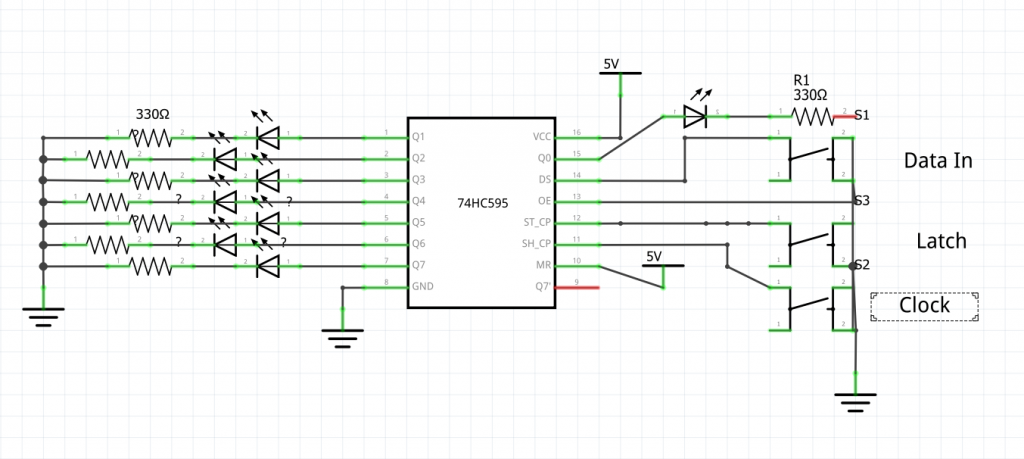
shiftBasic
Demonstrates the basic usage of the shiftOut() function to send a byte of data out to a shift register.
Click here to view code on GithubshiftFunction
We'll wrap our shiftOut() usage into a function we've written to keep things clean and concise.
Click here to view code on Github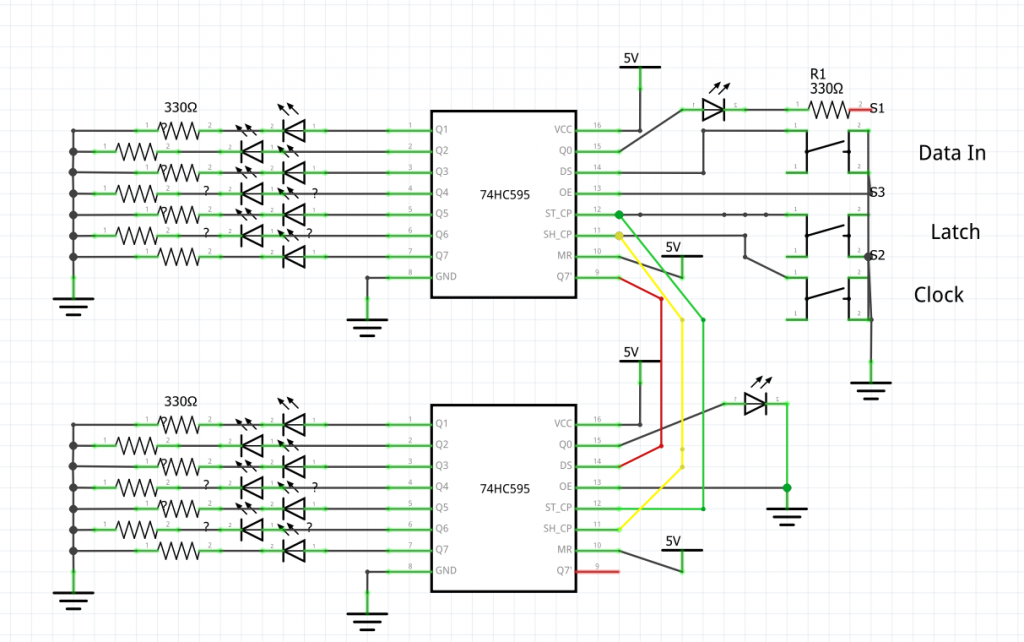
shiftInt
When writing out two bytes in a row, we can do two things. First, we can store both bytes as an int to keep them together by multiplying one of the bytes by 256. Second, we can delay latching the data in until both bytes are written using a new function called sendIntOut() that we've written.
Click here to view code on GithubLED Matrix and Gameplay
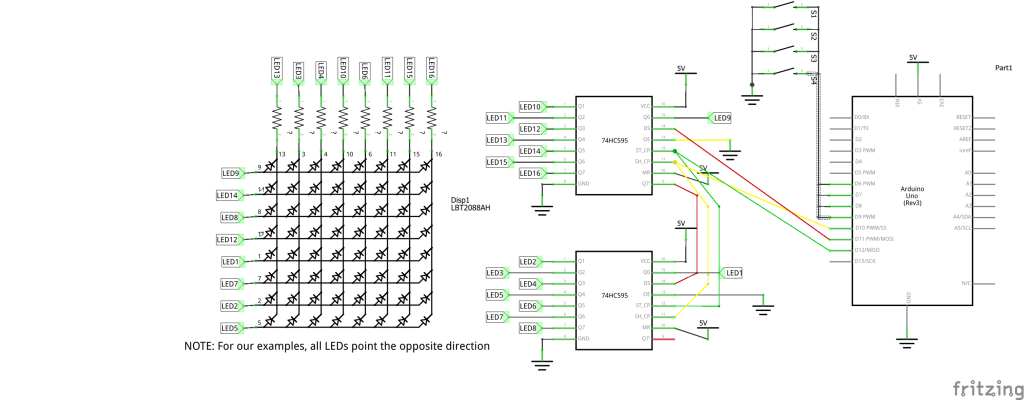
A_Pins.ino
Take our basic display code with shift registers as a starting point for our game code.
Click here to view code on GithubB_DisplayConstants.ino
Add some implementation-specific definitions for which bit on our shift register is connected to which Row/Column of the matrix. Includes a 2D array as well.
Click here to view code on GithubTwoD_Array.ino
A small bit of code to illustrate 2D arrays, since this is the first time we're seeing them.
Click here to view code on GithubC_Multiplex.ino
Create a full 8x8 LED display by sequentially running displaying each row of the display at high speed.
Click here to view code on GithubD_Gamestart.ino
We start to build the code for our actual game - thinking about how we'll represent our game within our 2D array data structure, and what variables we'll need to use to capture that data. Also we start thinking about initializing that data at the start of the game.
This is the point where our little Snake will start moving (in one direction) around the display!
Click here to view code on GithubE_Input.ino
We write some simple code to accept input from 4 directional buttons
Click here to view code on GithubF_Input2.ino
We tidy up the previous code using a new function that will set the direction of our snake for us.
Click here to view code on GithubG_Food.ino
Now the board has a piece of food on it for our snake to see (but not yet eat). We modify the gameplay and display code to account for this, and make use of the random() function to put the food in a random place each time.
Click here to view code on GithubH_Eatfood.ino
Now the snake can eat the food! We modify our gameplay code, and show how we can reuse the function that originally placed our food to give it a new spot on the board. We also add a function to allow our snake to grow by 1 each time we eat a piece of food.
Click here to view code on GithubI_Walls.ino
Up till now, the snake could slide right off the board at any time. We add a new function, isValidPosition(), to see if the snake should be able to move ot its next position before it actually moves tehre.
Click here to view code on GithubJ_Gameover.ino
A game isn't much of a game without a fail state. We'd add some checking so that if the snake collides with itself, it's gameover and we see a frowny face.
Click here to view code on GithubK_RandomStart.ino
Rather than start in the same place each time, we'll incorporate some more randomness to have the snake start in a random position. We'll use a loop structure that picks a starting position and direction for the snake, sees if it's valid, and if it's not, pick a new one!
Click here to view code on GithubL_Cornerfix.ino
One last pernicious bug - when our snake is in a corner, the input-logic allows it to "turn," but then it's still stuck. The new logic eliminates this edge case.
Click here to view code on GithubZ_Final.ino
The completed snake game! If you're interested in just browsing the completed code, you can find it here.
Click here to view code on Github